How to manually manage state in an LSTM network for stateful predictionA powerful and popular recurrent neural network is the long short-term model network or LSTM.It is widely used because the architecture overcomes the vanishing
and exposing gradient problem that plagues all recurrent neural
networks, allowing very large and very deep networks to be created.
Like other recurrent neural networks, LSTM networks maintain state,
and the specifics of how this is implemented in the Keras framework can
be confusing.
In this post, you will discover exactly how state is maintained in LSTM networks by the Keras deep learning library.
After reading this post, you will know:
- How to develop a naive LSTM network for a sequence prediction problem
- How to carefully manage state through batches and features with an LSTM network
- How to manually manage state in an LSTM network for stateful prediction
Kick-start your project with my new book Deep Learning With Python, including step-by-step tutorials and the Python source code files for all examples.
Let’s get started.
- Jul/2016: First published
- Update Mar/2017: Updated example for Keras 2.0.2, TensorFlow 1.0.1 and Theano 0.9.0
- Update Aug/2018: Updated examples for Python 3, updated stateful example to get 100% accuracy
- Update Mar/2019: Fixed typo in the stateful example
- Update Jul/2022: Updated for TensorFlow 2.x API
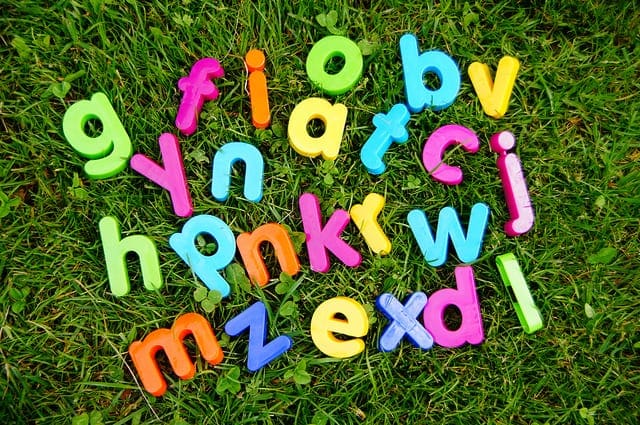
Understanding stateful LSTM recurrent neural networks in Python with Keras
Photo by Martin Abegglen, some rights reserved.
Problem Description: Learn the Alphabet
In this tutorial, you will develop and contrast a number of different LSTM recurrent neural network models.
The context of these comparisons will be a simple sequence prediction
problem of learning the alphabet. That is, given a letter of the
alphabet, it will predict the next letter of the alphabet.
This is a simple sequence prediction problem that, once understood,
can be generalized to other sequence prediction problems like time
series prediction and sequence classification.
Let’s prepare the problem with some Python code you can reuse from example to example.
First, let’s import all of the classes and functions you will use in this tutorial.
import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.utils import to_categorical |
Next, you can seed the random number generator to ensure that the results are the same each time the code is executed.
# fix random seed for reproducibility tf.random.set_seed(7) |
You can now define your dataset, the alphabet. You define the alphabet in uppercase characters for readability.
Neural networks model numbers, so you need to map the letters of the
alphabet to integer values. You can do this easily by creating a
dictionary (map) of the letter index to the character. You can also
create a reverse lookup for converting predictions back into characters
to be used later.
# define the raw dataset alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" # create mapping of characters to integers (0-25) and the reverse char_to_int = dict((c, i) for i, c in enumerate(alphabet)) int_to_char = dict((i, c) for i, c in enumerate(alphabet)) |
Now, You need to create your input and output pairs on which to
train your neural network. You can do this by defining an input
sequence length, then reading sequences from the input alphabet
sequence.
For example, use an input length of 1. Starting at the beginning of
the raw input data, you can read off the first letter “A” and the next
letter as the prediction “B.” You move along one character and repeat
until You reach a prediction of “Z.”
# prepare the dataset of input to output pairs encoded as integers seq_length = 1 dataX = [] dataY = [] for i in range(0, len(alphabet) - seq_length, 1): seq_in = alphabet[i:i + seq_length] seq_out = alphabet[i + seq_length] dataX.append([char_to_int[char] for char in seq_in]) dataY.append(char_to_int[seq_out]) print(seq_in, '->', seq_out) |
Also, print out the input pairs for sanity checking.
Running the code to this point will produce the following output,
summarizing input sequences of length 1 and a single output character.
A -> B B -> C C -> D D -> E E -> F F -> G G -> H H -> I I -> J J -> K K -> L L -> M M -> N N -> O O -> P P -> Q Q -> R R -> S S -> T T -> U U -> V V -> W W -> X X -> Y Y -> Z |
You need to reshape the NumPy array into a format expected by the LSTM networks, specifically [samples, time steps, features].
# reshape X to be [samples, time steps, features] X = np.reshape(dataX, (len(dataX), seq_length, 1)) |
Once reshaped, you can then normalize the input integers to the
range 0-to-1, the range of the sigmoid activation functions used by the
LSTM network.
# normalize X = X / float(len(alphabet)) |
Finally, you can think of this problem as a sequence
classification task, where each of the 26 letters represents a different
class. As such, you can convert the output (y) to a one-hot encoding
using the Keras built-in function to_categorical().
# one hot encode the output variable y = to_categorical(dataY) |
You are now ready to fit different LSTM models.
Need help with Deep Learning in Python?
Take my free 2-week email course and discover MLPs, CNNs and LSTMs (with code).
Click to sign-up now and also get a free PDF Ebook version of the course.
Naive LSTM for Learning One-Char to One-Char Mapping
Let’s start by designing a simple LSTM to learn how to predict the
next character in the alphabet, given the context of just one character.
You will frame the problem as a random collection of one-letter input
to one-letter output pairs. As you will see, this is a problematic
framing of the problem for the LSTM to learn.
Let’s define an LSTM network with 32 units and an output layer with a
softmax activation function for making predictions. Because this is a
multi-class classification problem, you can use the log loss function
(called “categorical_crossentropy” in Keras) and optimize the network using the ADAM optimization function.
The model is fit over 500 epochs with a batch size of 1.
# create and fit the model model = Sequential() model.add(LSTM(32, input_shape=(X.shape[1], X.shape[2]))) model.add(Dense(y.shape[1], activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) model.fit(X, y, epochs=500, batch_size=1, verbose=2) |
After you fit the model, you can evaluate and summarize the performance of the entire training dataset.
# summarize performance of the model scores = model.evaluate(X, y, verbose=0) print("Model Accuracy: %.2f%%" % (scores[1]*100)) |
You can then re-run the training data through the network and
generate predictions, converting both the input and output pairs back
into their original character format to get a visual idea of how well
the network learned the problem.
# demonstrate some model predictions for pattern in dataX: x = np.reshape(pattern, (1, len(pattern), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) result = int_to_char[index] seq_in = [int_to_char[value] for value in pattern] print(seq_in, "->", result) |
The entire code listing is provided below for completeness.
# Naive LSTM to learn one-char to one-char mapping import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.utils import to_categorical # fix random seed for reproducibility tf.random.set_seed(7) # define the raw dataset alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" # create mapping of characters to integers (0-25) and the reverse char_to_int = dict((c, i) for i, c in enumerate(alphabet)) int_to_char = dict((i, c) for i, c in enumerate(alphabet)) # prepare the dataset of input to output pairs encoded as integers seq_length = 1 dataX = [] dataY = [] for i in range(0, len(alphabet) - seq_length, 1): seq_in = alphabet[i:i + seq_length] seq_out = alphabet[i + seq_length] dataX.append([char_to_int[char] for char in seq_in]) dataY.append(char_to_int[seq_out]) print(seq_in, '->', seq_out) # reshape X to be [samples, time steps, features] X = np.reshape(dataX, (len(dataX), seq_length, 1)) # normalize X = X / float(len(alphabet)) # one hot encode the output variable y = to_categorical(dataY) # create and fit the model model = Sequential() model.add(LSTM(32, input_shape=(X.shape[1], X.shape[2]))) model.add(Dense(y.shape[1], activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) model.fit(X, y, epochs=500, batch_size=1, verbose=2) # summarize performance of the model scores = model.evaluate(X, y, verbose=0) print("Model Accuracy: %.2f%%" % (scores[1]*100)) # demonstrate some model predictions for pattern in dataX: x = np.reshape(pattern, (1, len(pattern), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) result = int_to_char[index] seq_in = [int_to_char[value] for value in pattern] print(seq_in, "->", result) |
Note: Your results may vary
given the stochastic nature of the algorithm or evaluation procedure,
or differences in numerical precision. Consider running the example a
few times and compare the average outcome.
Running this example produces the following output.
Model Accuracy: 84.00% ['A'] -> B ['B'] -> C ['C'] -> D ['D'] -> E ['E'] -> F ['F'] -> G ['G'] -> H ['H'] -> I ['I'] -> J ['J'] -> K ['K'] -> L ['L'] -> M ['M'] -> N ['N'] -> O ['O'] -> P ['P'] -> Q ['Q'] -> R ['R'] -> S ['S'] -> T ['T'] -> U ['U'] -> W ['V'] -> Y ['W'] -> Z ['X'] -> Z ['Y'] -> Z |
You can see that this problem is indeed difficult for the network to learn.
The reason is that the poor LSTM units do not have any context to
work with. Each input-output pattern is shown to the network in a random
order, and the state of the network is reset after each pattern (each
batch where each batch contains one pattern).
This is an abuse of the LSTM network architecture, treating it like a standard multilayer perceptron.
Next, let’s try a different framing of the problem to provide more sequence to the network from which to learn.
Naive LSTM for a Three-Char Feature Window to One-Char Mapping
A popular approach to adding more context to data for multilayer perceptrons is to use the window method.
This is where previous steps in the sequence are provided as
additional input features to the network. You can try the same trick to
provide more context to the LSTM network.
Here, you will increase the sequence length from 1 to 3, for example:
# prepare the dataset of input to output pairs encoded as integers seq_length = 3 |
This creates training patterns like this:
Each element in the sequence is then provided as a new input
feature to the network. This requires a modification of how the input
sequences are reshaped in the data preparation step:
# reshape X to be [samples, time steps, features] X = np.reshape(dataX, (len(dataX), 1, seq_length)) |
It also requires modifying how the sample patterns are reshaped when demonstrating predictions from the model.
x = np.reshape(pattern, (1, 1, len(pattern))) |
The entire code listing is provided below for completeness.
# Naive LSTM to learn three-char window to one-char mapping import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.utils import to_categorical # fix random seed for reproducibility tf.random.set_seed(7) # define the raw dataset alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" # create mapping of characters to integers (0-25) and the reverse char_to_int = dict((c, i) for i, c in enumerate(alphabet)) int_to_char = dict((i, c) for i, c in enumerate(alphabet)) # prepare the dataset of input to output pairs encoded as integers seq_length = 3 dataX = [] dataY = [] for i in range(0, len(alphabet) - seq_length, 1): seq_in = alphabet[i:i + seq_length] seq_out = alphabet[i + seq_length] dataX.append([char_to_int[char] for char in seq_in]) dataY.append(char_to_int[seq_out]) print(seq_in, '->', seq_out) # reshape X to be [samples, time steps, features] X = np.reshape(dataX, (len(dataX), 1, seq_length)) # normalize X = X / float(len(alphabet)) # one hot encode the output variable y = to_categorical(dataY) # create and fit the model model = Sequential() model.add(LSTM(32, input_shape=(X.shape[1], X.shape[2]))) model.add(Dense(y.shape[1], activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) model.fit(X, y, epochs=500, batch_size=1, verbose=2) # summarize performance of the model scores = model.evaluate(X, y, verbose=0) print("Model Accuracy: %.2f%%" % (scores[1]*100)) # demonstrate some model predictions for pattern in dataX: x = np.reshape(pattern, (1, 1, len(pattern))) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) result = int_to_char[index] seq_in = [int_to_char[value] for value in pattern] print(seq_in, "->", result) |
Note: Your results may vary
given the stochastic nature of the algorithm or evaluation procedure,
or differences in numerical precision. Consider running the example a
few times and compare the average outcome.
Running this example provides the following output.
Model Accuracy: 86.96% ['A', 'B', 'C'] -> D ['B', 'C', 'D'] -> E ['C', 'D', 'E'] -> F ['D', 'E', 'F'] -> G ['E', 'F', 'G'] -> H ['F', 'G', 'H'] -> I ['G', 'H', 'I'] -> J ['H', 'I', 'J'] -> K ['I', 'J', 'K'] -> L ['J', 'K', 'L'] -> M ['K', 'L', 'M'] -> N ['L', 'M', 'N'] -> O ['M', 'N', 'O'] -> P ['N', 'O', 'P'] -> Q ['O', 'P', 'Q'] -> R ['P', 'Q', 'R'] -> S ['Q', 'R', 'S'] -> T ['R', 'S', 'T'] -> U ['S', 'T', 'U'] -> V ['T', 'U', 'V'] -> Y ['U', 'V', 'W'] -> Z ['V', 'W', 'X'] -> Z ['W', 'X', 'Y'] -> Z |
You can see a slight lift in performance that may or may not be
real. This is a simple problem that you were still not able to learn
with LSTMs even with the window method.
Again, this is a misuse of the LSTM network by a poor framing of the
problem. Indeed, the sequences of letters are time steps of one feature
rather than one time step of separate features. You have given more
context to the network but not more sequence as expected.
In the next section, you will give more context to the network in the form of time steps.
Naive LSTM for a Three-Char Time Step Window to One-Char Mapping
In Keras, the intended use of LSTMs is to provide context in the form
of time steps, rather than windowed features like with other network
types.
You can take your first example and simply change the sequence length from 1 to 3.
Again, this creates input-output pairs that look like this:
ABC -> D BCD -> E CDE -> F DEF -> G |
The difference is that the reshaping of the input data takes
the sequence as a time step sequence of one feature rather than a single
time step of multiple features.
# reshape X to be [samples, time steps, features] X = np.reshape(dataX, (len(dataX), seq_length, 1)) |
This is the correct intended use of providing sequence context
to your LSTM in Keras. The full code example is provided below for
completeness.
# Naive LSTM to learn three-char time steps to one-char mapping import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.utils import to_categorical # fix random seed for reproducibility tf.random.set_seed(7) # define the raw dataset alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" # create mapping of characters to integers (0-25) and the reverse char_to_int = dict((c, i) for i, c in enumerate(alphabet)) int_to_char = dict((i, c) for i, c in enumerate(alphabet)) # prepare the dataset of input to output pairs encoded as integers seq_length = 3 dataX = [] dataY = [] for i in range(0, len(alphabet) - seq_length, 1): seq_in = alphabet[i:i + seq_length] seq_out = alphabet[i + seq_length] dataX.append([char_to_int[char] for char in seq_in]) dataY.append(char_to_int[seq_out]) print(seq_in, '->', seq_out) # reshape X to be [samples, time steps, features] X = np.reshape(dataX, (len(dataX), seq_length, 1)) # normalize X = X / float(len(alphabet)) # one hot encode the output variable y = to_categorical(dataY) # create and fit the model model = Sequential() model.add(LSTM(32, input_shape=(X.shape[1], X.shape[2]))) model.add(Dense(y.shape[1], activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) model.fit(X, y, epochs=500, batch_size=1, verbose=2) # summarize performance of the model scores = model.evaluate(X, y, verbose=0) print("Model Accuracy: %.2f%%" % (scores[1]*100)) # demonstrate some model predictions for pattern in dataX: x = np.reshape(pattern, (1, len(pattern), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) result = int_to_char[index] seq_in = [int_to_char[value] for value in pattern] print(seq_in, "->", result) |
Note: Your results may vary
given the stochastic nature of the algorithm or evaluation procedure,
or differences in numerical precision. Consider running the example a
few times and compare the average outcome.
Running this example provides the following output.
Model Accuracy: 100.00% ['A', 'B', 'C'] -> D ['B', 'C', 'D'] -> E ['C', 'D', 'E'] -> F ['D', 'E', 'F'] -> G ['E', 'F', 'G'] -> H ['F', 'G', 'H'] -> I ['G', 'H', 'I'] -> J ['H', 'I', 'J'] -> K ['I', 'J', 'K'] -> L ['J', 'K', 'L'] -> M ['K', 'L', 'M'] -> N ['L', 'M', 'N'] -> O ['M', 'N', 'O'] -> P ['N', 'O', 'P'] -> Q ['O', 'P', 'Q'] -> R ['P', 'Q', 'R'] -> S ['Q', 'R', 'S'] -> T ['R', 'S', 'T'] -> U ['S', 'T', 'U'] -> V ['T', 'U', 'V'] -> W ['U', 'V', 'W'] -> X ['V', 'W', 'X'] -> Y ['W', 'X', 'Y'] -> Z |
You can see that the model learns the problem perfectly as evidenced by the model evaluation and the example predictions.
But it has learned a simpler problem. Specifically, it has learned to
predict the next letter from a sequence of three letters in the
alphabet. It can be shown any random sequence of three letters from the
alphabet and predict the next letter.
It can not actually enumerate the alphabet. It’s possible a larger
enough multilayer perception network might be able to learn the same
mapping using the window method.
The LSTM networks are stateful. They should be able to learn the
whole alphabet sequence, but by default, the Keras implementation resets
the network state after each training batch.
LSTM State within a Batch
The Keras implementation of LSTMs resets the state of the network after each batch.
This suggests that if you had a batch size large enough to hold all
input patterns and if all the input patterns were ordered sequentially,
the LSTM could use the context of the sequence within the batch to
better learn the sequence.
You can demonstrate this easily by modifying the first example for
learning a one-to-one mapping and increasing the batch size from 1 to
the size of the training dataset.
Additionally, Keras shuffles the training dataset before each
training epoch. To ensure the training data patterns remain sequential,
you can disable this shuffling.
model.fit(X, y, epochs=5000, batch_size=len(dataX), verbose=2, shuffle=False) |
The network will learn the mapping of characters using the
within-batch sequence, but this context will not be available to the
network when making predictions. You can evaluate both the ability of
the network to make predictions randomly and in sequence.
The full code example is provided below for completeness.
# Naive LSTM to learn one-char to one-char mapping with all data in each batch import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.utils import to_categorical from tensorflow.keras.preprocessing.sequence import pad_sequences # fix random seed for reproducibility tf.random.set_seed(7) # define the raw dataset alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" # create mapping of characters to integers (0-25) and the reverse char_to_int = dict((c, i) for i, c in enumerate(alphabet)) int_to_char = dict((i, c) for i, c in enumerate(alphabet)) # prepare the dataset of input to output pairs encoded as integers seq_length = 1 dataX = [] dataY = [] for i in range(0, len(alphabet) - seq_length, 1): seq_in = alphabet[i:i + seq_length] seq_out = alphabet[i + seq_length] dataX.append([char_to_int[char] for char in seq_in]) dataY.append(char_to_int[seq_out]) print(seq_in, '->', seq_out) # convert list of lists to array and pad sequences if needed X = pad_sequences(dataX, maxlen=seq_length, dtype='float32') # reshape X to be [samples, time steps, features] X = np.reshape(dataX, (X.shape[0], seq_length, 1)) # normalize X = X / float(len(alphabet)) # one hot encode the output variable y = to_categorical(dataY) # create and fit the model model = Sequential() model.add(LSTM(16, input_shape=(X.shape[1], X.shape[2]))) model.add(Dense(y.shape[1], activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) model.fit(X, y, epochs=5000, batch_size=len(dataX), verbose=2, shuffle=False) # summarize performance of the model scores = model.evaluate(X, y, verbose=0) print("Model Accuracy: %.2f%%" % (scores[1]*100)) # demonstrate some model predictions for pattern in dataX: x = np.reshape(pattern, (1, len(pattern), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) result = int_to_char[index] seq_in = [int_to_char[value] for value in pattern] print(seq_in, "->", result) # demonstrate predicting random patterns print("Test a Random Pattern:") for i in range(0,20): pattern_index = np.random.randint(len(dataX)) pattern = dataX[pattern_index] x = np.reshape(pattern, (1, len(pattern), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) result = int_to_char[index] seq_in = [int_to_char[value] for value in pattern] print(seq_in, "->", result) |
Note: Your results may vary
given the stochastic nature of the algorithm or evaluation procedure,
or differences in numerical precision. Consider running the example a
few times and compare the average outcome.
Running the example provides the following output.
Model Accuracy: 100.00% ['A'] -> B ['B'] -> C ['C'] -> D ['D'] -> E ['E'] -> F ['F'] -> G ['G'] -> H ['H'] -> I ['I'] -> J ['J'] -> K ['K'] -> L ['L'] -> M ['M'] -> N ['N'] -> O ['O'] -> P ['P'] -> Q ['Q'] -> R ['R'] -> S ['S'] -> T ['T'] -> U ['U'] -> V ['V'] -> W ['W'] -> X ['X'] -> Y ['Y'] -> Z Test a Random Pattern: ['T'] -> U ['V'] -> W ['M'] -> N ['Q'] -> R ['D'] -> E ['V'] -> W ['T'] -> U ['U'] -> V ['J'] -> K ['F'] -> G ['N'] -> O ['B'] -> C ['M'] -> N ['F'] -> G ['F'] -> G ['P'] -> Q ['A'] -> B ['K'] -> L ['W'] -> X ['E'] -> F |
As expected, the network is able to use the within-sequence
context to learn the alphabet, achieving 100% accuracy on the training
data.
Importantly, the network can make accurate predictions for the next
letter in the alphabet for randomly selected characters. Very
impressive.
Stateful LSTM for a One-Char to One-Char Mapping
You have seen that you can break up the raw data into fixed-size
sequences and that this representation can be learned by the LSTM but
only to learn random mappings of 3 characters to 1 character.
You have also seen that you can pervert the batch size to offer more sequence to the network, but only during training.
Ideally, you want to expose the network to the entire sequence and
let it learn the inter-dependencies rather than you defining those
dependencies explicitly in the framing of the problem.
You can do this in Keras by making the LSTM layers stateful and
manually resetting the state of the network at the end of the epoch,
which is also the end of the training sequence.
This is truly how the LSTM networks are intended to be used.
You first need to define your LSTM layer as stateful. In so doing,
you must explicitly specify the batch size as a dimension on the input
shape. This also means that when you evaluate the network or make
predictions, you must also specify and adhere to this same batch size.
This is not a problem now as you are using a batch size of 1. This could
introduce difficulties when making predictions when the batch size is
not one, as predictions will need to be made in the batch and the
sequence.
batch_size = 1 model.add(LSTM(50, batch_input_shape=(batch_size, X.shape[1], X.shape[2]), stateful=True)) |
An important difference in training the stateful LSTM is that
you manually train it one epoch at a time and reset the state after each
epoch. You can do this in a for loop. Again, do not shuffle the input,
preserving the sequence in which the input training data was created.
for i in range(300): model.fit(X, y, epochs=1, batch_size=batch_size, verbose=2, shuffle=False) model.reset_states() |
As mentioned, you specify the batch size when evaluating the performance of the network on the entire training dataset.
# summarize performance of the model scores = model.evaluate(X, y, batch_size=batch_size, verbose=0) model.reset_states() print("Model Accuracy: %.2f%%" % (scores[1]*100)) |
Finally, you can demonstrate that the network has indeed
learned the entire alphabet. You can seed it with the first letter “A,”
request a prediction, feed the prediction back in as an input, and
repeat the process all the way to “Z.”
# demonstrate some model predictions seed = [char_to_int[alphabet[0]]] for i in range(0, len(alphabet)-1): x = np.reshape(seed, (1, len(seed), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) print(int_to_char[seed[0]], "->", int_to_char[index]) seed = [index] model.reset_states() |
You can also see if the network can make predictions starting from an arbitrary letter.
# demonstrate a random starting point letter = "K" seed = [char_to_int[letter]] print("New start: ", letter) for i in range(0, 5): x = np.reshape(seed, (1, len(seed), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) print(int_to_char[seed[0]], "->", int_to_char[index]) seed = [index] model.reset_states() |
The entire code listing is provided below for completeness.
# Stateful LSTM to learn one-char to one-char mapping import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.utils import to_categorical # fix random seed for reproducibility tf.random.set_seed(7) # define the raw dataset alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" # create mapping of characters to integers (0-25) and the reverse char_to_int = dict((c, i) for i, c in enumerate(alphabet)) int_to_char = dict((i, c) for i, c in enumerate(alphabet)) # prepare the dataset of input to output pairs encoded as integers seq_length = 1 dataX = [] dataY = [] for i in range(0, len(alphabet) - seq_length, 1): seq_in = alphabet[i:i + seq_length] seq_out = alphabet[i + seq_length] dataX.append([char_to_int[char] for char in seq_in]) dataY.append(char_to_int[seq_out]) print(seq_in, '->', seq_out) # reshape X to be [samples, time steps, features] X = np.reshape(dataX, (len(dataX), seq_length, 1)) # normalize X = X / float(len(alphabet)) # one hot encode the output variable y = to_categorical(dataY) # create and fit the model batch_size = 1 model = Sequential() model.add(LSTM(50, batch_input_shape=(batch_size, X.shape[1], X.shape[2]), stateful=True)) model.add(Dense(y.shape[1], activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) for i in range(300): model.fit(X, y, epochs=1, batch_size=batch_size, verbose=2, shuffle=False) model.reset_states() # summarize performance of the model scores = model.evaluate(X, y, batch_size=batch_size, verbose=0) model.reset_states() print("Model Accuracy: %.2f%%" % (scores[1]*100)) # demonstrate some model predictions seed = [char_to_int[alphabet[0]]] for i in range(0, len(alphabet)-1): x = np.reshape(seed, (1, len(seed), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) print(int_to_char[seed[0]], "->", int_to_char[index]) seed = [index] model.reset_states() # demonstrate a random starting point letter = "K" seed = [char_to_int[letter]] print("New start: ", letter) for i in range(0, 5): x = np.reshape(seed, (1, len(seed), 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) print(int_to_char[seed[0]], "->", int_to_char[index]) seed = [index] model.reset_states() |
Note: Your results may vary
given the stochastic nature of the algorithm or evaluation procedure,
or differences in numerical precision. Consider running the example a
few times and compare the average outcome.
Running the example provides the following output.
Model Accuracy: 100.00% A -> B B -> C C -> D D -> E E -> F F -> G G -> H H -> I I -> J J -> K K -> L L -> M M -> N N -> O O -> P P -> Q Q -> R R -> S S -> T T -> U U -> V V -> W W -> X X -> Y Y -> Z New start: K K -> B B -> C C -> D D -> E E -> F |
You can see that the network has memorized the entire alphabet
perfectly. It used the context of the samples themselves and learned
whatever dependency it needed to predict the next character in the
sequence.
You can also see that if you seed the network with the first letter, it can correctly rattle off the rest of the alphabet.
You can also see that it has only learned the full alphabet sequence
and from a cold start. When asked to predict the next letter from “K,”
it predicts “B” and falls back into regurgitating the entire alphabet.
To truly predict “K,” the state of the network would need to be
warmed up and iteratively fed the letters from “A” to “J.” This reveals
that you could achieve the same effect with a “stateless” LSTM by
preparing training data like this:
---a -> b --ab -> c -abc -> d abcd -> e |
Here, the input sequence is fixed at 25 (a-to-y to predict z), and patterns are prefixed with zero padding.
Finally, this raises the question of training an LSTM network using
variable length input sequences to predict the next character.
LSTM with Variable-Length Input to One-Char Output
In the previous section, you discovered that the Keras “stateful”
LSTM was really only a shortcut to replaying the first n-sequences but
didn’t really help us learn a generic model of the alphabet.
In this section, you will explore a variation of the “stateless” LSTM
that learns random subsequences of the alphabet and an effort to build a
model that can be given arbitrary letters or subsequences of letters
and predict the next letter in the alphabet.
First, you are changing the framing of the problem. To simplify, you
will define a maximum input sequence length and set it to a small value
like 5 to speed up training. This defines the maximum length of
subsequences of the alphabet that will be drawn for training. In
extensions, this could just be set to the full alphabet (26) or longer
if you allow looping back to the start of the sequence.
You also need to define the number of random sequences to create—in
this case, 1000. This, too, could be more or less. It’s likely fewer
patterns are actually required.
# prepare the dataset of input to output pairs encoded as integers num_inputs = 1000 max_len = 5 dataX = [] dataY = [] for i in range(num_inputs): start = np.random.randint(len(alphabet)-2) end = np.random.randint(start, min(start+max_len,len(alphabet)-1)) sequence_in = alphabet[start:end+1] sequence_out = alphabet[end + 1] dataX.append([char_to_int[char] for char in sequence_in]) dataY.append(char_to_int[sequence_out]) print(sequence_in, '->', sequence_out) |
Running this code in the broader context will create input patterns that look like the following:
PQRST -> U W -> X O -> P OPQ -> R IJKLM -> N QRSTU -> V ABCD -> E X -> Y GHIJ -> K |
The input sequences vary in length between 1 and max_len and therefore require zero padding. Here, use left-hand-side (prefix) padding with the Keras built-in pad_sequences() function.
X = pad_sequences(dataX, maxlen=max_len, dtype='float32') |
The trained model is evaluated on randomly selected input
patterns. This could just as easily be new randomly generated sequences
of characters. This could also be a linear sequence seeded with “A” with
outputs fed back in as single character inputs.
The full code listing is provided below for completeness.
# LSTM with Variable Length Input Sequences to One Character Output import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.utils import to_categorical from tensorflow.keras.preprocessing.sequence import pad_sequences # fix random seed for reproducibility np.random.seed(7) tf.random.set_seed(7) # define the raw dataset alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" # create mapping of characters to integers (0-25) and the reverse char_to_int = dict((c, i) for i, c in enumerate(alphabet)) int_to_char = dict((i, c) for i, c in enumerate(alphabet)) # prepare the dataset of input to output pairs encoded as integers num_inputs = 1000 max_len = 5 dataX = [] dataY = [] for i in range(num_inputs): start = np.random.randint(len(alphabet)-2) end = np.random.randint(start, min(start+max_len,len(alphabet)-1)) sequence_in = alphabet[start:end+1] sequence_out = alphabet[end + 1] dataX.append([char_to_int[char] for char in sequence_in]) dataY.append(char_to_int[sequence_out]) print(sequence_in, '->', sequence_out) # convert list of lists to array and pad sequences if needed X = pad_sequences(dataX, maxlen=max_len, dtype='float32') # reshape X to be [samples, time steps, features] X = np.reshape(X, (X.shape[0], max_len, 1)) # normalize X = X / float(len(alphabet)) # one hot encode the output variable y = to_categorical(dataY) # create and fit the model batch_size = 1 model = Sequential() model.add(LSTM(32, input_shape=(X.shape[1], 1))) model.add(Dense(y.shape[1], activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) model.fit(X, y, epochs=500, batch_size=batch_size, verbose=2) # summarize performance of the model scores = model.evaluate(X, y, verbose=0) print("Model Accuracy: %.2f%%" % (scores[1]*100)) # demonstrate some model predictions for i in range(20): pattern_index = np.random.randint(len(dataX)) pattern = dataX[pattern_index] x = pad_sequences([pattern], maxlen=max_len, dtype='float32') x = np.reshape(x, (1, max_len, 1)) x = x / float(len(alphabet)) prediction = model.predict(x, verbose=0) index = np.argmax(prediction) result = int_to_char[index] seq_in = [int_to_char[value] for value in pattern] print(seq_in, "->", result) |
Note: Your results may vary
given the stochastic nature of the algorithm or evaluation procedure,
or differences in numerical precision. Consider running the example a
few times and compare the average outcome.
Running this code produces the following output:
Model Accuracy: 98.90% ['Q', 'R'] -> S ['W', 'X'] -> Y ['W', 'X'] -> Y ['C', 'D'] -> E ['E'] -> F ['S', 'T', 'U'] -> V ['G', 'H', 'I', 'J', 'K'] -> L ['O', 'P', 'Q', 'R', 'S'] -> T ['C', 'D'] -> E ['O'] -> P ['N', 'O', 'P'] -> Q ['D', 'E', 'F', 'G', 'H'] -> I ['X'] -> Y ['K'] -> L ['M'] -> N ['R'] -> T ['K'] -> L ['E', 'F', 'G'] -> H ['Q'] -> R ['Q', 'R', 'S'] -> T |
You can see that although the model did not learn the alphabet
perfectly from the randomly generated subsequences, it did very well.
The model was not tuned and might require more training, a larger
network, or both (an exercise for the reader).
This is a good natural extension to the “all sequential input examples in each batch”
alphabet model learned above in that it can handle ad hoc queries, but
this time of arbitrary sequence length (up to the max length).
Summary
In this post, you discovered LSTM recurrent neural networks in Keras and how they manage state.
Specifically, you learned:
- How to develop a naive LSTM network for one-character to one-character prediction
- How to configure a naive LSTM to learn a sequence across time steps within a sample
- How to configure an LSTM to learn a sequence across samples by manually managing state
Do you have any questions about managing an LSTM state or this post?
Ask your questions in the comment, and I will do my best to answer.
No comments:
Post a Comment