How to Develop an Encoder-Decoder Model with Attention in Keras
The encoder-decoder architecture for recurrent neural networks is proving to be powerful on a host of sequence-to-sequence prediction problems in the field of natural language processing such as machine translation and caption generation.
Attention is a mechanism that addresses a limitation of the encoder-decoder architecture on long sequences, and that in general speeds up the learning and lifts the skill of the model no sequence to sequence prediction problems.
In this tutorial, you will discover how to develop an encoder-decoder recurrent neural network with attention in Python with Keras.
After completing this tutorial, you will know:
- How to design a small and configurable problem to evaluate encoder-decoder recurrent neural networks with and without attention.
- How to design and evaluate an encoder-decoder network with and without attention for the sequence prediction problem.
- How to robustly compare the performance of encoder-decoder networks with and without attention.
Kick-start your project with my new book Long Short-Term Memory Networks With Python, including step-by-step tutorials and the Python source code files for all examples.
Let’s get started.
- Note May/2020: The underlying APIs have changed and this tutorial may no longer be current. You may require older versions of Keras and TensorFlow, e.g. Keras 2 and TF 1.
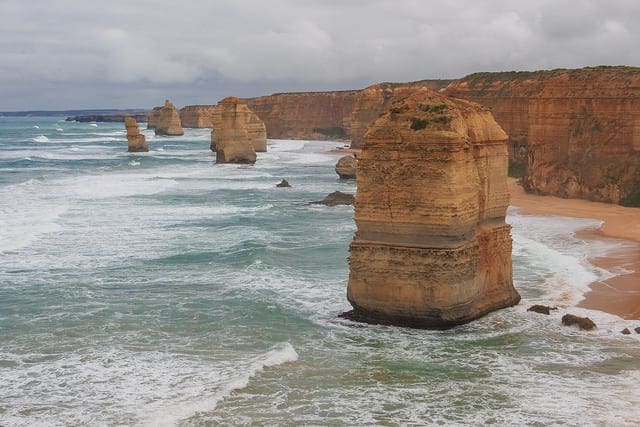
How to Develop an Encoder-Decoder Model with Attention for Sequence-to-Sequence Prediction in Keras
Photo by Angela and Andrew, some rights reserved.
Tutorial Overview
This tutorial is divided into 6 parts; they are:
- Encoder-Decoder with Attention
- Test Problem for Attention
- Encoder-Decoder without Attention
- Custom Keras Attention Layer
- Encoder-Decoder with Attention
- Comparison of Models
Python Environment
This tutorial assumes you have a Python 3 SciPy environment installed.
You must have Keras (2.0 or higher) installed with either the TensorFlow or Theano backend.
The tutorial also assumes you have scikit-learn, Pandas, NumPy, and Matplotlib installed.
If you need help with your environment, see this post:
Encoder-Decoder with Attention
The encoder-decoder model for recurrent neural networks is an architecture for sequence-to-sequence prediction problems.
It is comprised of two sub-models, as its name suggests:
- Encoder: The encoder is responsible for stepping through the input time steps and encoding the entire sequence into a fixed length vector called a context vector.
- Decoder: The decoder is responsible for stepping through the output time steps while reading from the context vector.
A problem with the architecture is that performance is poor on long input or output sequences. The reason is believed to be because of the fixed-sized internal representation used by the encoder.
Attention is an extension to the architecture that addresses this limitation. It works by first providing a richer context from the encoder to the decoder and a learning mechanism where the decoder can learn where to pay attention in the richer encoding when predicting each time step in the output sequence.
For more on attention in the encoder-decoder architecture, see the posts:
- Attention in Long Short-Term Memory Recurrent Neural Networks
- How Does Attention Work in Encoder-Decoder Recurrent Neural Networks
Test Problem for Attention
Before we develop models with attention, we will first define a contrived scalable test problem that we can use to determine whether attention is providing any benefit.
In this problem, we will generate sequences of random integers as input and matching output sequences comprised of a subset of the integers in the input sequence.
For example, an input sequence might be [1, 6, 2, 7, 3] and the expected output sequence might be the first two random integers in the sequence [1, 6].
We will define the problem such that the input and output sequences are the same length and pad the output sequences with “0” values as needed.
First, we need a function to generate sequences of random integers. We will use the Python randint() function to generate random integers between 0 and a maximum value and use this range as the cardinality for the problem (e.g. the number of features or an axis of difficulty).
The function generate_sequence() below will generate a random sequence of integers to a fixed length and with the specified cardinality.
Running this example generates a sequence of 5 time steps where each value in the sequence is a random integer between 0 and 49.
Next, we need a function to one hot encode the discrete integer values into binary vectors.
If a cardinality of 50 is used, then each integer will be represented by a 50-element vector of 0 values and 1 in the index of the specified integer value.
The one_hot_encode() function below will one hot encode a given sequence of integers.
We also need to be able to decode an encoded sequence. This will be needed to turn a prediction from the model or an encoded expected sequence back into a sequence of integers we can read and evaluate.
The one_hot_decode() function below will decode a one hot encoded sequence back into a sequence of integers.
We can test out these operations in the example below.
Running the example first prints a randomly generated sequence, then the one hot encoded version, then finally the decoded sequence again.
Finally, we need a function that can create input and output pairs of sequences to train and evaluate a model.
The function below named get_pair() will return one input and output sequence pair given a specified input length, output length, and cardinality. Both input and output sequences are the same length, the length of the input sequence, but the output sequence will be taken as the first n characters of the input sequence and padded with zero values to the required length.
The sequences of integers are then encoded then reshaped into a 3D format required for the recurrent neural network, with the dimensions: samples, time steps, and features. In this case, samples is always 1 as we are only generating one input-output pair, the time steps is the input sequence length and features is the cardinality of each time step.
We can put this all together and demonstrate the data preparation code.
Running the example generates a single input-output pair and prints the shape of both arrays.
The generated pair is then printed in a decoded form where we can see that the first two integers of the sequence are reproduced in the output sequence followed by a padding of zero values.
Encoder-Decoder Without Attention
In this section, we will develop a baseline in performance on the problem with an encoder-decoder model without attention.
We will fix the problem definition at input and output sequences of 5 time steps, the first 2 elements of the input sequence in the output sequence and a cardinality of 50.
We can develop a simple encoder-decoder model in Keras by taking the output from an encoder LSTM model, repeating it n times for the number of timesteps in the output sequence, then using a decoder to predict the output sequence.
For more detail on how to define an encoder-decoder architecture in Keras, see the post:
We will configure the encoder and decoder with the same number of units, in this case 150. We will use the efficient Adam implementation of gradient descent and optimize the categorical cross entropy loss function, given that the problem is technically a multi-class classification problem.
The configuration for the model was found after a little trial and error and is by no means optimized.
The code for an encoder-decoder architecture in Keras is listed below.
We will train the model on 5,000 random input-output pairs of integer sequences.
Once trained, we will evaluate the model on 100 new randomly generated integer sequences and only mark a prediction correct when the entire output sequence matches the expected value.
Finally, we will print 10 examples of expected output sequences and sequences predicted by the model.
Putting all of this together, the complete example is listed below.
Running this example will not take long, perhaps a few minutes on the CPU, no GPU is required.
Note: Your results may vary given the stochastic nature of the algorithm or evaluation procedure, or differences in numerical precision. Consider running the example a few times and compare the average outcome.
The accuracy of the model was reported at just under 20%.
We can see from the sample outputs that the model does get one number in the output sequence correct for most or all cases, and only struggles with the second number. All zero padding values are predicted correctly.
Custom Keras Attention Layer
Now we need to add attention to the encoder-decoder model.
At the time of writing, Keras does not have the capability of attention built into the library, but it is coming soon.
Until attention is officially available in Keras, we can either develop our own implementation or use an existing third-party implementation.
To speed things up, let’s use an existing third-party implementation.
Zafarali Ahmed an intern at Datalogue developed a custom layer for Keras that provides support for attention, presented in a post titled “How to Visualize Your Recurrent Neural Network with Attention in Keras” in 2017 and GitHub project called “keras-attention“.
The custom attention layer is called AttentionDecoder and is available in the custom_recurrents.py file in the GitHub project. We can reuse this code under the GNU Affero General Public License v3.0 license of the project.
A copy of the custom layer is listed below for completeness. Copy it and paste it into a new and separate file in your current working directory called ‘attention_decoder.py‘.
We can make use of this custom layer in our projects by importing it as follows:
The layer implements attention as described by Bahdanau, et al. in their paper “Neural Machine Translation by Jointly Learning to Align and Translate.”
The code is explained well in the original post and linked to both the LSTM and attention equations.
A limitation of this implementation is that it must output sequences that are the same length as the input sequences, the specific limitation that the encoder-decoder architecture was designed to overcome.
Importantly, the new layer manages both the repeating of the decoding as performed by the second LSTM, as well as the softmax output for the model as was performed by the Dense output layer in the encoder-decoder model without attention. This greatly simplifies the code for the model.
It is important to note that the custom layer is built upon the Recurrent layer in Keras, which, at the time of writing, is marked as legacy code, and presumably will be removed from the project at some point.
Encoder-Decoder With Attention
Now that we have an implementation of attention that we can use, we can develop an encoder-decoder model with attention for our contrived sequence prediction problem.
The model with the attention layer is defined below. We can see that the layer handles some of the machinery of the encoder-decoder model itself, making defining the model simpler.
That’s it. The rest of the example is the same.
The complete example is listed below.
Running the example prints the skill of the model on 100 randomly generated input-output pairs.
Note: Your results may vary given the stochastic nature of the algorithm or evaluation procedure, or differences in numerical precision. Consider running the example a few times and compare the average outcome.
With the same resources and same amount of training, the model with attention performs much better.
Spot-checking some sample outputs and predicted sequences, we can see very few errors, even in cases when there is a zero value in the first two elements.
Comparison of Models
Although we are getting better results from the model with attention, the results were reported from a single run of each model.
In this case, we seek a more robust finding by repeating the evaluation of each model multiple times and reporting the average performance over those runs. For more information on this robust approach to evaluating neural network models, see the post:
We can define a function to create each type of model, as follows.
We can then define a function to fit and evaluate the accuracy of a fit model and return the accuracy score.
Putting this together, we can repeat the process of creating, training, and evaluating each type of model multiple times and reporting the mean accuracy over the repeats. To keep running times down, we will repeat each model evaluation 10 times, although if you have the resources, you could increase this to 30 or 100 times.
The complete example is listed below.
Note: Your results may vary given the stochastic nature of the algorithm or evaluation procedure, or differences in numerical precision. Consider running the example a few times and compare the average outcome.
Running this example prints the accuracy for each model repeat to give you an idea of the progress of the run.
We can see that even averaged over 10 runs, the attention model still shows better performance than the encoder-decoder model without attention, 23.10% vs 95.70%.
A good extension to this evaluation would be to capture the model loss each epoch for each model, take the average, and compare how the loss changes over time for the architecture with and without attention.
I expect that this trace would show attention achieving better skill much faster and sooner than the non-attentional model, further highlighting the benefit of the approach.
Further Reading
This section provides more resources on the topic if you are looking to go deeper.
- Attention in Long Short-Term Memory Recurrent Neural Networks
- How Does Attention Work in Encoder-Decoder Recurrent Neural Networks
- Encoder-Decoder Long Short-Term Memory Networks
- How to Evaluate the Skill of Deep Learning Models
- How to Visualize Your Recurrent Neural Network with Attention in Keras, 2017.
- keras-attention GitHub Project
- Neural Machine Translation by Jointly Learning to Align and Translate, 2015.
Summary
In this tutorial, you discovered how to develop an encoder-decoder recurrent neural network with attention in Python with Keras.
Specifically, you learned:
- How to design a small and configurable problem to evaluate encoder-decoder recurrent neural networks with and without attention.
- How to design and evaluate an encoder-decoder network with and without attention for the sequence prediction problem.
- How to robustly compare the performance of encoder-decoder networks with and without attention.
Do you have any questions?
Ask your questions in the comments below and I will do my best to answer
No comments:
Post a Comment